Login Screen with validations
In this article, we will discuss Ionic3 and angular 2 validations. How we can display validation messages.
Let's create a new login page in the application. You can either add all the pages (.ts .html and .scss file manually) or you can create by running the command mention below.
ionic generate page Login.
it will automatically add a page in your application as shown in below snapshot.
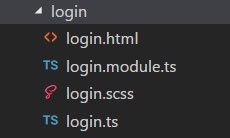
it will automatically add a page in your application as shown in below snapshot.
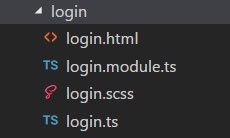
Login.ts
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
2) In Constructor, add form builder as a parameter as shown in below snapshot.
3) Declare account as any object and add 2 properties "email" and "password"
4) For Email, compose validators (Required and Email type) as shown below.
export class LoginPage {
account:any;
constructor(public navCtrl: NavController, public navParams: NavParams,public alertCtrl: AlertController, formBuilder: FormBuilder) {
this.account = formBuilder.group({
email: ['', Validators.compose([Validators.required,Validators.email])],
password: ['',Validators.compose([Validators.required,Validators.maxLength(5)])]
});
}
}
Construct HTML Form
1) Open Login.html file, pass account model in formGroup
2) formControlName to textbox
3) [class.invalid], if you want to give a red border on validation. This is custom class mention below
4) With the help of hasError property, you can check the exact error.
5) Disable login button, if page is not valid [disabled]="!account.valid"
account.controls.email.hasError('required')
account.controls.email.hasError('email')
account.controls.email.hasError('maxlength')
account.controls.email.hasError('minlength')
account.controls.email.hasError('pattern')
<form [formGroup]="account" (submit)="doLogin()">
<ion-list>
<ion-item>
<ion-label floating>Email</ion-label>
<ion-input type="text" formControlName="email"
[class.invalid]="!account.controls.email.valid && account.controls.email.dirty">
</ion-input>
</ion-input>
</ion-item>
<p class="errorMessage"
*ngIf="!account.controls.email.hasError('required') && account.controls.email.hasError('email') && account.controls.email.dirty)">
Please enter a valid
email.
</p>
<p class="errorMessage"
*ngIf="account.controls.email.hasError('required')
&& (account.controls.email.dirty )">Please enter email
</p>
<ion-item>
<ion-label floating>Password</ion-label>
<ion-input type="password" formControlName="password" [class.invalid]="!account.controls.password.valid && account.controls.password.dirty">
</ion-input>
</ion-item>
<p class="errorMessage" *ngIf="!account.controls.password.hasError('required') && account.controls.password.hasError('maxlength')
&& (account.controls.password.dirty)">Max length should be
5.
</p>
<p class="errorMessage"
*ngIf="account.controls.password.hasError('required') && (account.controls.password.dirty)">
Please enter password
</p>
<div padding>
<button ion-button color="dark" block type="submit" [disabled]="!account.valid">Login</button>
</div>
</ion-list>
</form>
Styling the Form
1) Open Login.scss file.
page-login {
.invalid {
border-bottom: 1px solid #FF6153;
}
.errorMessage
{
color: red;
font-size: 10px;
margin-left: 20px;
}
}
Submit Button Functionality
Login button functionality, I've checked with static email and password.
If both are matched then redirect to Next Page otherwise show an alert message.
Open Login.ts file and paste the below method in it.
doLogin() {
if(this.account.value.email =="test@gmail.com" && this.account.value.password=="test")
{
this.navCtrl.push(TabsPage);
}
else{
let alert = this.alertCtrl.create({
title: 'Message',
subTitle:'Invalid Login',
buttons: ['OK']
});
alert.present();
}
}
For alert, you need to import Alert Controller on top of the page.
For navigation, we need the Nav Controller.
import { IonicPage, NavController, NavParams } from 'ionic-angular';
import { AlertController } from 'ionic-angular';
The Output would be like below screenshot.
Button will be enabled only, if we will input valid text in the textbox.
That's all about the article. Thanks for reading. If you have any question related to this article. you can mention in comments box below.